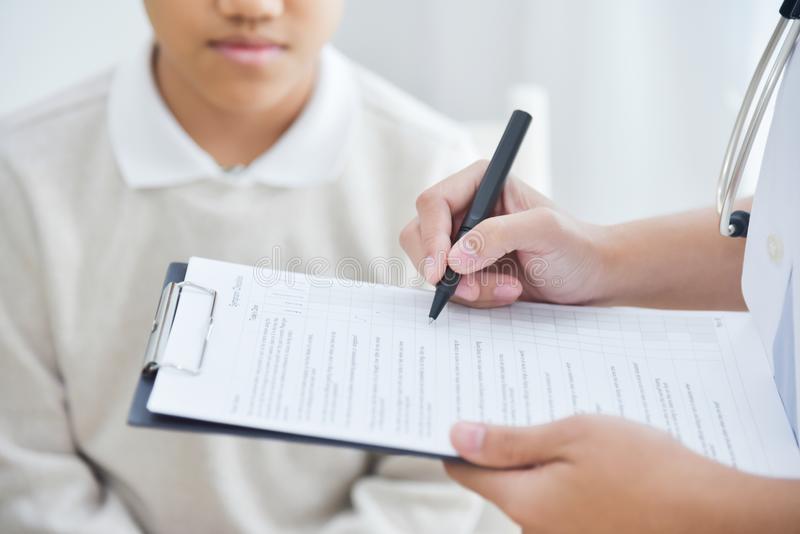
Angular Cheat Sheet for Web Development
Angular is defined as a front-end web development framework that is specifically used to build single-page web applications, which are also called SPAs. This platform uses TypeScript as the main programming language. The angular framework itself is coded in the TypeScript language. Angular is considered as a dogmatic framework. While developing apps with Angular, you must bear it in mind that it specifies a certain style and lays down a specific set of rules that development professionals need to learn, follow, and stick to. Hence, you need to learn Angular and its different modules, components, and tools which are necessary to make an app while using Angular.
As a beginner, you may hear about different versions of Angular, which is more likely to confuse you. Some versions include AngularJS, Angular 2, Angular 4, Angular 5, Angular 6, Angular 7, Angular 8, and recently in February 2020, and Angular 9 was introduced. There are only two different frameworks of Angular, which are; AngularJS and Angular.
This article will let you explore the Angular Cheat Sheet, which can be used as a quick reference guide while learning the language. Let’s go through the main concepts, commands, utilities, and other basics of Angular.
Angular CLI
The angular command-line interface, also called Angular CLI, is a very powerful and useful tool that enables developers to perform a variety of actions in an Angular project through the use of simple commands. The CLI takes care of everything, ranging from supporting a brand-new Angular project to building the project for production.
Furthermore, CLI also allows developers to debug the application during development. It’s done through locally using a development server which overlooks the source files for changes. Once the change is made in any of the files, by default, it automatically recompiles the source code files and refreshes the app in the browser.
The CLI tool can be used directly in a command shell, or indirectly via an interactive UI, such as Angular Console.
Here are some ways to use the Angular CLI:
Command |
Description |
ng help |
This command gives you options to get help about any utility command. |
ng version |
The command prints the version info of the currently running CLI. |
ng new |
This command generates a new Angular project with the given name. |
ng serve |
This command Spins up the local development server and launches the web app locally in the browser. Moreover, this command also considers more parameters, like port and open. The server automatically rebuilds the app and reloads the page when any of the source files are changed. |
ng build |
Complies and builds the Angular app into a directory that can be deployed on the webserver. |
ng generate |
It is used to generate modules, services, components, classes, providers, pipes, etc. |
Setup
Command |
Description |
npm install -g @angular/CLI |
Globally Install Angular CLI |
New Application
Command |
Description |
ng new best-practices --dry-run |
just simulate ng new |
ng new best-practices --skip-install |
skip install means don't run npm install |
ng new best-practices --prefix best |
set prefix to best |
ng new --help |
check available command list |
Lint
Lint enables you to check and make sure that code is free of code smells or bad formatting.
Command |
Description |
ng lint my-app --help |
Checks available command list |
ng lint my-app --format stylish |
Formats code |
ng lint my-app --fix |
Fixes code bad formatting |
ng lint my-app |
Shows warnings |
Blue Prints
Command |
Description |
ng g c my-component --flat true |
Don't create a new folder for this component |
--inline-template (-t) |
Will the template be in .ts file? |
--inline-style (-s) |
Will the style be in .ts file? |
--spec |
Generates spec or not? |
--prefix |
Assigns own prefix |
ng g d directive-name |
Directive creation |
ng g s service-name |
Service creation |
ng g cl models/customer |
Customer class creation in the model's folder |
ng g I models/person |
Create interface creation in the model's folder |
ng g e models/gender |
Create ENUM gender creation in the model's folder |
ng g p init-caps |
Create pipe creation |
Building & Serving
Command |
Description |
ng build |
Build apps to dist. folder |
ng build --aot |
Builds app without a code which is not needed |
ng build --prod |
Builds for production |
ng serve -o |
Serves with browser opening |
ng serve --live-reload |
Reloads as per changes |
ng serve -ssl |
Serving while using SSL |
Adding New Capabilities
Command |
Description |
ng add @angular/material |
Adds Angular material into the project |
ng g @angular/material:material-nav --name nav |
Creates material navigation module |
Components and Templates
Components are considered as the most basic UI building blocks of any Angular app. An app built in Angular contains a chain of elements.
Example
import { Component } from '@angular/core';
@Component({
// component attributes
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.less']
})
export class AppComponent {
title = 'my-cats-training';
}
Component Attributes
Attribute |
Description |
changeDetection |
The change detection plan to use for this component. |
viewProviders |
Defines the set of injectable entities which are visible to its view DOM children |
module |
The module ID of the element which contains the component |
encapsulation |
An encapsulation rule used for the template and CSS styles |
interpolation |
Overrules the built-in encapsulation start and end delimiters |
entryComponents |
A collection of components which must be compiled along with this component. |
preserveWhitespaces |
If True, then preserve, or if False then removes, potentially redundant whitespace characters from the compiled template. |