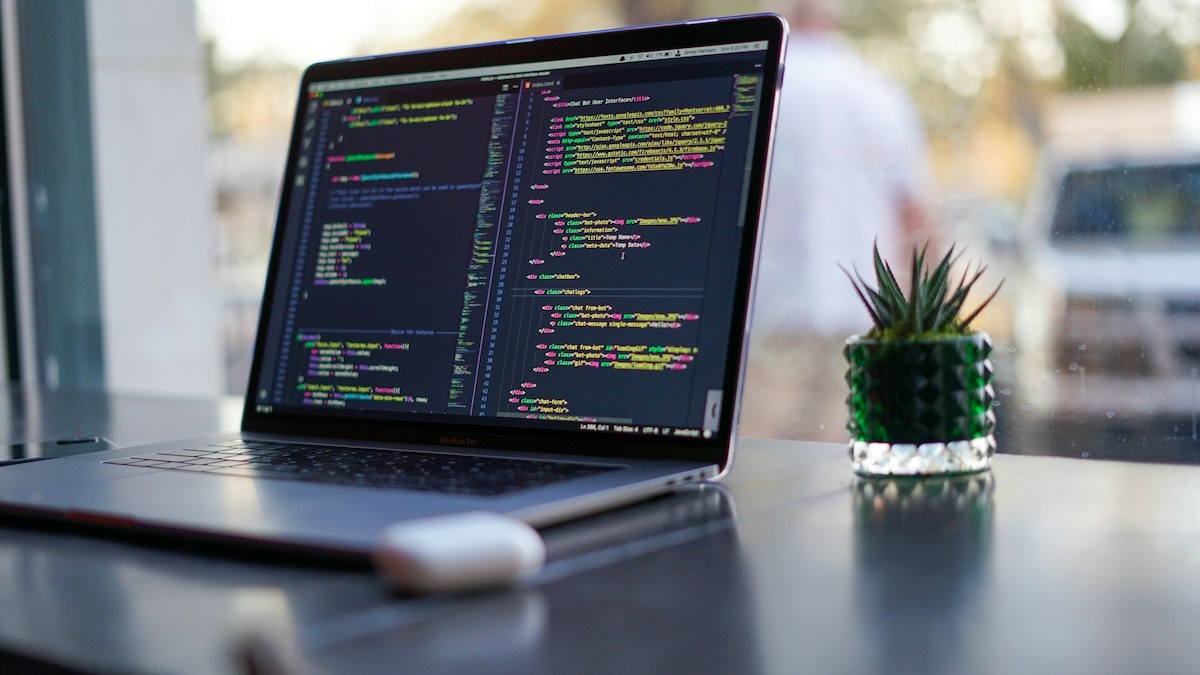
Mastering Object-Oriented Programming in Python: Applications and Key Concepts
Object-oriented programming (OOP) is a powerful paradigm that organizes code around objects, enhancing organization, reusability, and maintainability.
OOP represents a fundamental shift in the way software is designed and structured. Instead of thinking solely in terms of procedures and functions, OOP encourages developers to model their programs around real-world objects and the interactions between them. These objects encapsulate both data (attributes) and behavior (methods), creating a self-contained unit that can be easily understood and manipulated.
OOP stands as a cornerstone for developing complex applications, primarily due to its intrinsic ability to foster modular and scalable code. By breaking down intricate systems into self-contained objects, OOP enables developers to manage and expand their codebases with remarkable ease. OOP also aligns software design with the real world, allowing developers to model entities from the environment and encapsulate data securely within these objects. This approach not only enhances code organization; it also ensures the robustness and efficiency of the resulting software.
Object-oriented programming is an understandably complicated concept, one best explained in a full OOP Python course or begin with the foundations in a software engineering bootcamp. Below, we’ll explain some basic OOP concepts and their use in comprehensive code.
The Python Object
The Python programming language embraces a comprehensive object model where everything, be it a basic integer or an intricate data structure, is treated as an object. Within this model, each object possesses three essential attributes: a distinctive identity, a specific type that dictates its behavior, and a value that encapsulates its data. This foundational concept underscores Python's versatility and consistency, as it allows developers to interact with a wide range of entities in a uniform and object-oriented manner.
x = 42
print(type(x)) # <class 'int'>
Understanding the identity, type, and value of objects in Python is incredibly important. Each forms part of the foundation of the programming language itself:
Identity
The identity of an object in Python is a unique identifier, often represented as the object's memory address. This identity ensures that no two objects are the same, even if they have the same value. Recognizing the identity of objects is crucial for tasks like comparing objects for equality (using is), tracking object references, and managing memory efficiently. It helps prevent unintended side effects when working with mutable objects, as you can identify if two variables reference the same object or distinct ones.
Type
An object's type defines its behavior and the operations that can be performed on it. Python is dynamically typed, meaning the type of an object is determined at runtime. Understanding the type of an object is vital for making informed decisions in your code, such as selecting appropriate methods or operations. It enables you to handle objects differently based on their types, facilitating polymorphism and adaptability in your programs.
Value
The value of an object is the actual data it holds. It represents the content and characteristics of the object. Recognizing the value of objects is essential for data manipulation and processing. You need to access and modify the values of objects to achieve your programming goals. Whether you're working with integers, strings, lists, or custom classes, understanding the values of objects allows you to extract information, transform data, and generate meaningful results.
y = "Hello"
print(id(y)) # Memory address of the object
print(type(y)) # <class 'str'>
print(y) # Hello
Essential Constructs in Python OOP
Python offers several vital constructs that serve as the cornerstone of object-oriented programming.
Classes serve as blueprints for creating objects. A class defines a data structure that includes attributes to hold data and methods to operate on that data. These attributes can represent various types of data, and methods encapsulate the behavior and actions related to that data. By defining the data structure within a class, you establish a clear and organized way to represent and manage the data related to objects of that class.
A class will also define both the structure and behavior of objects. The structure is determined by the attributes that represent the data, and the behavior is defined by the methods that operate on that data. This dual role of classes allows you to create objects that not only hold data but also exhibit specific behaviors or actions associated with that data.
class Dog:
def __init__(self, name):
self.name = name
def bark(self):
print(f"{self.name} says woof!")
Objects in Python are instances of classes. When you create an object, you're essentially instantiating a specific instance of the data structure and behavior defined by a class. This relationship between classes and objects is at the core of object-oriented programming in Python.
Objects are tangible entities that embody the attributes and methods specified by their parent class. They represent real-world entities or abstract concepts and encapsulate both data (attributes) and functionality (methods). Each object has a unique identity, type, and value, distinguishing it from other objects, even if they belong to the same class.
Objects are not limited to representing physical entities; they can represent abstract concepts or data structures as well. For instance, a ‘BankAccount’ class can be used to create objects that represent individual bank accounts, each with its balance, account number, and transaction methods.
my_dog = Dog("Buddy")
my_dog.bark() # Buddy says woof!
Attributes are essentially variables that store data within a class or an object. They can represent various types of data, such as integers, strings, lists, or more complex data structures. Attributes define the characteristics or properties of an object, and they can have default values or be initialized when an object is created.
Attributes are crucial for representing the state of an object, allowing you to store and retrieve data associated with that object. They provide the means to keep track of an object's properties and maintain its integrity throughout its lifecycle.
class Circle:
def __init__(self, radius):
self.radius = radius
def calculate_area(self):
return 3.14 * self.radius ** 2
my_circle = Circle(5)
print(my_circle.calculate_area()) # 78.5
Methods are essentially functions that are associated with a class. They encapsulate behavior or actions that objects of that class can perform. These actions can range from simple operations, like calculating a value or displaying information, to more complex tasks, such as interacting with external systems, processing data, or modifying the object's state.
Inheritance is a powerful concept in object-oriented programming that facilitates code reuse and extensibility. It allows one class (the subclass or derived class) to inherit attributes and methods from another class (the superclass or base class). This mechanism enables developers to create specialized classes that build upon the functionality of existing classes, promoting efficient and organized code development. It also promotes code reuse by allowing developers to create new classes that inherit attributes and methods from existing classes. This means you can define a base class with common attributes and methods that multiple specialized classes can utilize without the need to rewrite the same code.
class Cat(Dog):
def purr(self):
print(f"{self.name} says purr!")
my_cat = Cat("Whiskers")
my_cat.bark() # Whiskers says woof!
my_cat.purr() # Whiskers says purr!
Encapsulation is a crucial concept in object-oriented programming that provides a mechanism for bundling data and methods into a single unit known as a class. It serves several fundamental purposes and offers numerous benefits in software development.
One of the primary advantages of encapsulation is that it provides a level of data protection and access control. By encapsulating data within a class, you can control how that data is accessed and modified. In most object-oriented programming languages, including Python, you can define access modifiers such as private, protected, and public to restrict or allow access to certain attributes and methods. This control ensures data integrity and prevents unauthorized modification.
Encapsulation hides the internal details of an object. For instance, encapsulating a car's details:
class Car:
def __init__(self, make, model):
self.__make = make # Encapsulated attribute
self.__model = model
def get_details(self):
return f"{self.__make} {self.__model}"
my_car = Car("Toyota", "Camry")
print(my_car.get_details()) # Toyota Camry
Polymorphism is a fundamental concept in object-oriented programming that enables flexibility when working with common base classes. It allows objects of different classes to be treated as objects of a shared base class, fostering code flexibility and extensibility.
At the core of polymorphism is the idea that objects can take on multiple forms. When objects of different classes inherit from a common base class or implement a common interface, they share a certain set of attributes and methods defined by that base class or interface. As a result, these objects can be treated interchangeably when they exhibit similar behavior or provide a consistent interface.
def animal_sound(animal):
animal.make_sound()
class Dog:
def make_sound(self):
print("Woof!")
class Cat:
def make_sound(self):
print("Meow!")
my_dog = Dog()
my_cat = Cat()
animal_sound(my_dog) # Woof!
animal_sound(my_cat) # Meow!
Class Inheritance
Class inheritance allows a new class, known as the subclass or derived class, to inherit attributes and methods from an existing class, known as the superclass or base class. The subclass inherits the structure and behavior defined in the superclass, making it a natural extension of the base class.
Class inheritance allows a new class, known as the subclass or derived class, to inherit attributes and methods from an existing class, known as the superclass or base class. The subclass inherits the structure and behavior defined in the superclass, making it a natural extension of the base class.
Subclasses have the flexibility to extend or override the functionality inherited from the base class. This means that while they inherit the common features from the superclass, they can introduce their own attributes and methods or provide their implementations for existing methods. This customization allows you to create specialized classes that cater to specific requirements while benefiting from the foundational structure provided by the base class.
class Vehicle:
def __init__(self, brand):
self.brand = brand
def display_info(self):
print(f"Brand: {self.brand}")
class Car(Vehicle):
def __init__(self, brand, model):
super().__init__(brand)
self.model = model
def display_info(self):
super().display_info()
print(f"Model: {self.model}")
my_car = Car("Toyota", "Camry")
my_car.display_info()
# Output:
# Brand: Toyota
# Model: Camry
In the example above, ‘Car’ is a derived class that inherits from the base class ‘Vehicle’. The super() function is used to call methods from the parent class.
Extending or overriding functionality in derived classes. Here, ‘ElectricCar’ extends the functionality of ‘Car’ and overrides the display_info method.
class ElectricCar(Car):
def __init__(self, brand, model, battery_capacity):
super().__init__(brand, model)
self.battery_capacity = battery_capacity
def display_info(self):
super().display_info()
print(f"Battery Capacity: {self.battery_capacity} kWh")
my_electric_car = ElectricCar("Tesla", "Model S", 100)
my_electric_car.display_info()
# Output:
# Brand: Tesla
# Model: Model S
# Battery Capacity: 100 kWh
The Constructor/Destructor Magic Methods
The __init__ constructor method is a fundamental and special feature in Python classes, serving as the initiation point when an object is created from a class. Its primary purpose is to initialize the attributes of the object, allowing you to set up the initial state of the instance.
When you create an object from a class, you often want to provide specific initial values for its attributes. This is where the __init__ method comes into play. By defining this method within your class, you can specify the initial values for the object's attributes, ensuring that the object starts with the desired state.
In Python, you can define default values for parameters in the __init__ method, making certain attributes optional during object creation. This allows you to create objects with partial attribute sets or provide default values when necessary.
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
person = Person("John", 25)
print(person.name) # John
print(person.age) # 25
The __del__ destructor method is another special method in Python classes, but its usage and significance differ from that of the __init__ constructor method. While the __init__ method is called when an object is created to initialize its attributes, the __del__ method is called just before an object is destroyed, allowing for cleanup operations to be performed.
The primary purpose of the __del__ destructor method is to give you an opportunity to perform cleanup operations before the object is removed from memory. It can be helpful for releasing resources or performing tasks that should be carried out before an object is no longer accessible.
It's essential to note that relying on the __del__ destructor is not always necessary in Python. Python employs automatic garbage collection to reclaim memory and resources when objects are no longer in use. Most of the time, the garbage collector efficiently handles memory management and resource cleanup without the need for explicit destructor methods.
class FileHandler:
def __init__(self, filename):
self.filename = filename
print(f"File {self.filename} opened")
def __del__(self):
print(f"File {self.filename} closed")
file_handler = FileHandler("example.txt")
# File example.txt opened
# The object will be automatically deleted at the end of the script or when no longer referenced.
The __del__ method is not always guaranteed to be called promptly, so it's often better to use context managers (‘with’ statement) for resource cleanup.
Get Started in Python OOP Today
There's a lot to learn in the world of Python OOP:
- Classes serve as templates for creating objects, which are instances of those classes.
- These objects encapsulate both data and behavior, promoting a structured and organized approach to coding.
- Inheritance allows one class to inherit attributes and methods from another, fostering code reuse and specialization.
- Encapsulation combines data and methods into a single unit, enhancing data security and controlled access.
- Polymorphism enables flexibility by allowing objects of different classes to be treated as if they belong to a common base class, simplifying code design and extensibility.
- Constructors, represented by the special method "init," initialize object attributes when they are created, while destructors, indicated by "del," are used for cleanup operations before an object is removed from memory.
The versatility of Object-Oriented Programming (OOP) extends far beyond the examples we've explored. OOP is not limited to specific domains; rather, it's a programming paradigm that can be applied to a wide range of scenarios across various industries and fields. Whether you're developing software for finance, healthcare, gaming, scientific research, or any other domain, OOP offers a robust framework for creating scalable, maintainable, and efficient code.
In the world of finance, for instance, OOP can be employed to model complex financial instruments, transactions, and portfolios. Similarly, in healthcare, OOP can facilitate the modeling of patient records, medical procedures, and healthcare systems. For those in the gaming industry, OOP is a valuable tool for developing interactive and immersive gaming experiences. Scientific research benefits from OOP as well, as it enables the creation of objects and classes to represent scientific phenomena, experiments, and simulations, aiding researchers in their data analysis and modeling efforts.
One of the best ways to learn OOP is through a Python Object Oriented Programming Fundamentals course — where you’ll discover principles in the Python Object, essential constructs, and class inheritance. You might also consider a software engineering bootcamp, one that familiarizes you with the front-end and back-end development skills you’ll need for long-term success in the IT space.
If you’re just getting started in Python, you’ll also need a Python Programming: Introduction course, such as the one offered by QuickStart, where you’ll learn basic applications, directory management, and conditional statements and loops.